[TUTORIAL] Hooking Android bytecode with Frida
Info
Hi. Today I’m going to show you how to hook Android bytecode using Frida. For this article I’ve prepared an Android application with simple function that simulates genering hashes in Android games. Function is called „calculateCRC32” and it takes String as an input parameter, it calculates CRC32 checksum from that string as a long value and then it converts this value to String to be able to print it on your screen.
To start making progress with this tutorial, you need some things first:
- A phone with Android OS – my choice is Realme 8i with Android 11
- A Frida client installed on your PC. I have Frida v16.6.6. You can check Frida version with „–version” parameter
- A Frida server installed on your android phone. It has to be the same (or similar) version as Frida client to avoid errors. In my case it’s frida-server-16.2.4-android-arm64
- Total Commander installed on your PC – just to do some text searching
- Notepad++ installed on your PC – just to read SMALI files
- ADB Platform Tools – just to run frida server with „adb shell” command
- APK Tool to decompile APK file to Android’s bytecode. I’m using APK Toolkit v1.3 (by 0xd00d)
- APK file to decompile and hook – I’ve prepared a file called „crc32_example.apk” for this tutorial.
Download it below:
Once you collect/install all those things, you should be able to start with the next chapter.
Testing the App
Download „crc32_example.apk” and move it to your phone’s internal storage or SD card. Then install APK, launch it and verify what shows up on your screen:
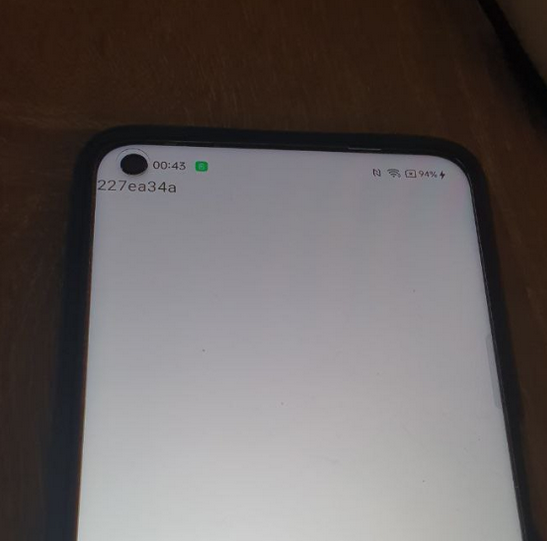
In this test app you can only see blank screen with small text „227ea34a”. This is our calculated checksum and our goal here is to find out what was the input string that was passed to crc function.
Decompiling the code
You need to know which function you want to hook. I already told in the first chapter you that the name of the function is „calculateCRC32„, but when you will be doing your own research, you will have to figure it out yourself. So let’s decompile the APK and search for this function:
Open APK Toolkit, set a path to the APK file and then decompile with a button:
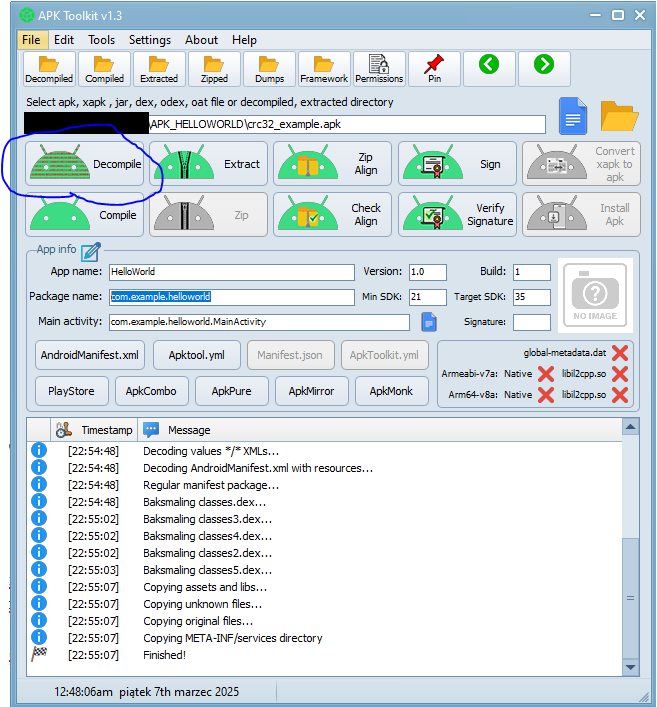
Now go to the decompiled directory and search for „calculateCRC32” with total commander:
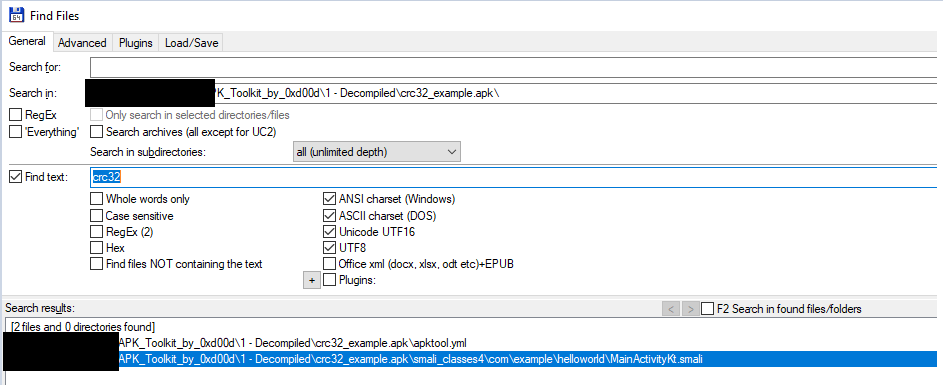
One result has been found in „smali_classes” directory, which is good in our case. So let’s go to specified directory and let’s open this SMALI file in Notepad++.
When you’ll search for CRC32 function in Notepad++, you will find definition on line 380:
Also we can get the class name from the same file. It’s in the first line:

So we were able to find out what we want to hook. Now let’s get to hooking itself.
Hooking with Frida
To hook properly, you need to create a Frida script. Create a file called „frida_android_bytecode_hook.js” and save it on your hard drive.
Now fill the script with below code and save it again:
Java.perform(function() {
var targetClass = Java.use("com.example.helloworld.MainActivityKt");
targetClass.calculateCRC32.overload("java.lang.String").implementation = function(arg) {
console.log("[*] Hooked: calculateCRC32");
console.log("Argument: " + arg);
var originalResult = this.calculateCRC32(arg);
console.log("Original result: " + originalResult);
var modifiedResult = "HOOKED_RESULT";
console.log("Modified result: " + modifiedResult);
return modifiedResult;
};
});
Script is complete, so now you have to make sure that your Frida server is running on your Android phone.
You can run the server with below command:
adb shell "su -c /data/local/tmp/frida-server-16.2.4-android-arm64 &"
The last step is to run the script itself with below command:
frida -U -f com.example.helloworld -l frida_android_bytecode_hook.js
Now you can just observe the result:
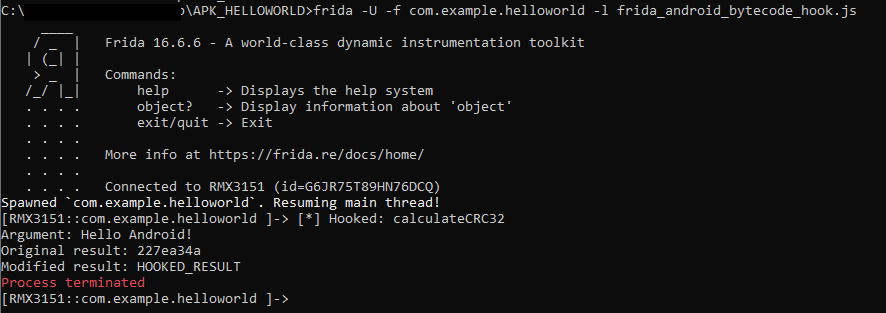
And this is how it will look on your phone:
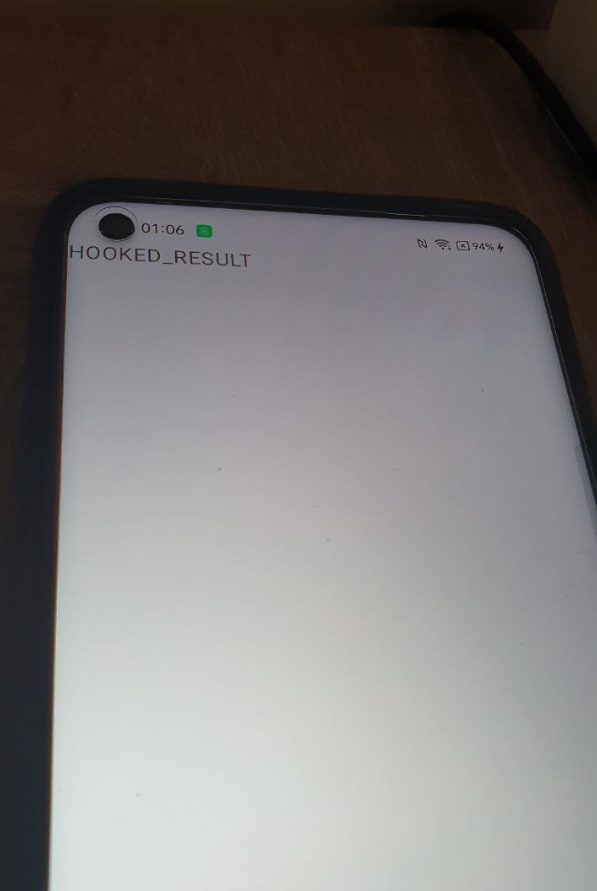
Summary
So let’s break it down what happend here. We were able to locate the function that we wanted to hook. We found out what was the class storing that function and the decompiled SMALI file that was holding that information to prepare our hook.
Then we’ve prepared a script and we have spawned an Android process with our hook.
Frida hook showed us few things:
- Argument for CRC32 function is „Hello Android!„. That was the string that we were looking for, so we’ve achieved our goal.
- CRC function result is „227ea34a„. We’ve already knew that from the initial application run, but this just confirms that we’ve set our hook in a good place.
- Changed result is „HOOKED_RESULT”. This is modified text that we were able to show on our phone’s screen.
Finally, you can confirm your result with some online CRC32 calculator, e.g. CyberChef:
https://gchq.github.io/CyberChef
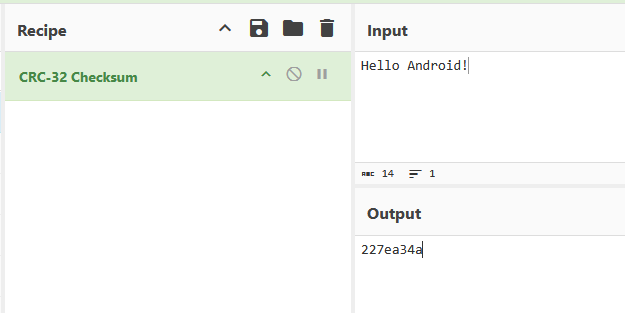
And that’s it for this tutorial. Happy hooking.